Trial Exe using vb.net
http://www.advancedinstaller.com/user-guide/tutorial-licensing.html
This tutorial will guide you in creating a VB application with licensing and trial support using Advanced Installer.
This walkthrough is designed to help you gain a better understanding of how the licensing feature in Advanced Installer works and how you can implement a licensing component into your own project. Throughout this example we'll create a simple Visual Basic project using Visual Studio 2008.
Our sample application itself will have a simple form but you should find sufficient comments in the underlying code to assist you in implementing this feature into any of your own projects. The project will include a very simple 'About' form which will display the licensed state of the application.
Because the nature of this walkthrough is to help you understand the licensing process in its entirety we are going to flip backwards and forwards between Advanced Installer and the application code. It's assumed that you are familiar with the basics of using Visual Studio so these will not be gone into.
So with our Visual Basic project completed as far as we wish to go at present we'll create a quick build in Advanced Installer using the Import Visual Studio Project wizard and then we will set the licensing options.

We will later add the code that will verify the trial period and registration key.

Complete the wizard by selecting the Visual Basic solution created earlier and you will find your application in Files and Folders page.

Save the project by using
the toolbar button and choose the file name and
the destination folder.

Click the toolbar button to add a licensing library to your
project.

The licensing library will be placed into the Application Folder and you can see it in the Files and Folders page. Note that the name of the library file corresponds with the trial configuration name.
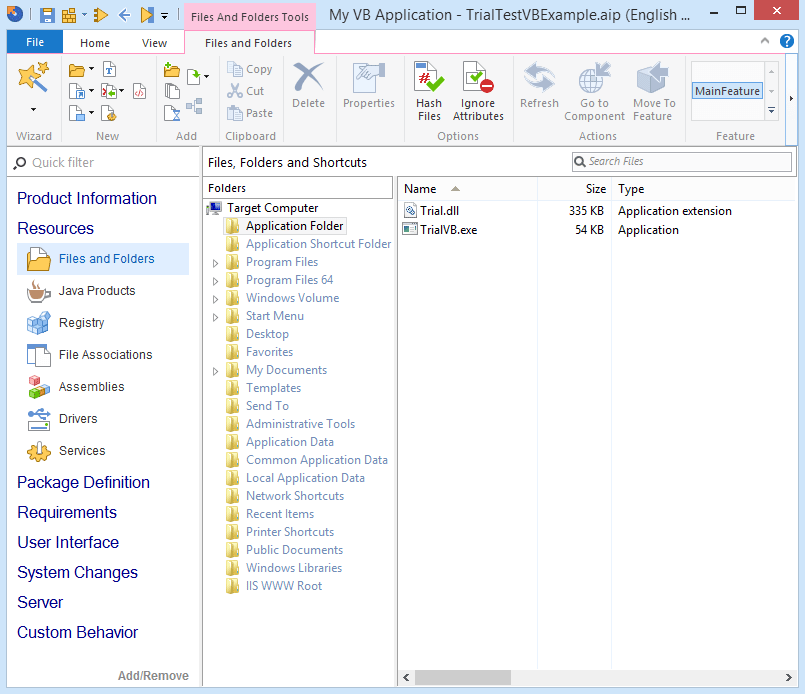
The licensing library name will be used in the application
source code so it's better to leave it in the same folder as the
application.
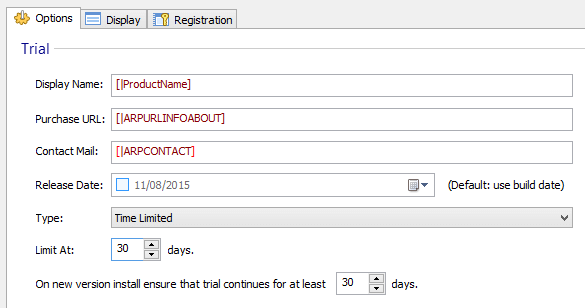
Display Name - the application name which will be used in the Licensing user interface. By default it will be the Product Name you entered during the import wizard or changed in the Product Details page.
Purchase Url - your Web Site page that handles purchases. By default it will be the "Product URL" specified in the Product Details page.
Advanced Installer supports several types of trial periods:
Set the trial period quantity into the "Limit At" field to a small value, let's say 10. This way the trial will expire after 10 runs or after 10 days since installation.
Finally in the "On new version install extend to" field we will set the number of days and uses that we are prepared to extend the trial by when a new version of the application is installed. Set this value to 5 because the user already had some time to try the application in the previous version.
Lets not choose to support trial extension options to keep thinks simpler.
Now we should specify which kind of application will use the licensing library.

There are several types of library

Here we can control what our registration wizard will look like and the frequency with which it is displayed during our trial period.
Please note that the display frequency percentage is applied to
the number of times that the application is opened and does not
strictly adhere to the choice that you made on the previous tab
about the type of trial that you would like to implement. The
default is set to 30 which means that you will on average see the
trial dialog every third time that you open the application.
We will enable the “Show the trial message at first
run” option since it is meant for applications that
display a license agreement or a welcome message at the first
run.
You can change the default “Banner Image” and “Dialog Image” but keep in mind that they will only be used on operating systems earlier than Windows Vista.
Into the Form1.vb source file add the following code snippets:
Don't forget to replace the Library Key from the code snippet
with the one from the Advanced Installer project > Licensing > Registration
page.
You will not be able to test the application until you install the
MSI package. After that you can run the application from its install
location. If you want to run the application from Visual Studio you
will have to copy the "Trial.dll" library from the install location to
the "bin" > "Debug" and/or "bin" > "Release" folders under the Visual
Studio project folder.
Click on the
toolbar button and a “Build
Project” dialog will appear showing you the build
evolution.
Once the build is complete,
click on the toolbar button. A setup wizard will
appear that will guide you through the install process.
Congratulations! You have successfully created your licensed application. By default, the application file will be installed under C:\Program Files\Your Company\. Browse to that folder in Windows Explorer and run the application.
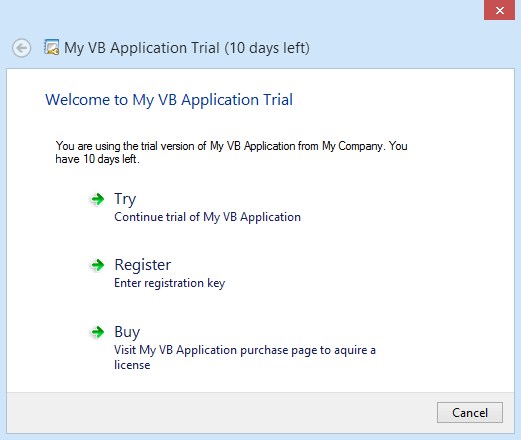

Click the button and the VB form should appear. In the “About” dialog the trial message should be displayed.
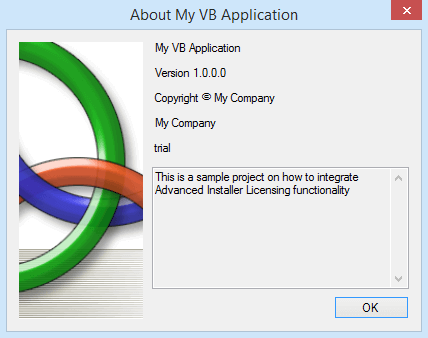
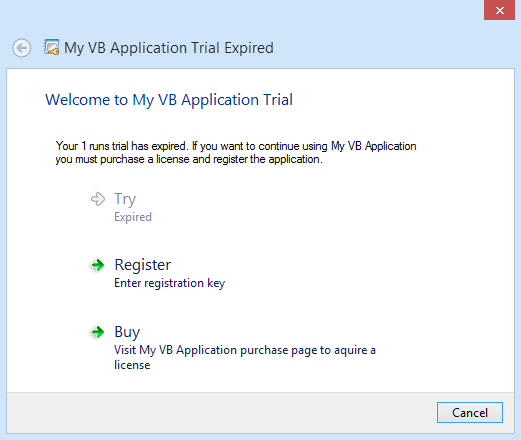
At this point the user needs to register the application since we didn't enable the trial extension option. Registration codes can be generated from the Advanced Installer -> Licensing -> Registration page by using the “Generate Registration Keys” link.
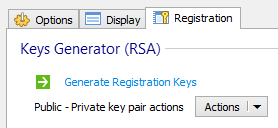
In the “Generate Registry Keys” dialog generate any number of keys you want. The keys will be saved into a file from where you can easily pick one when you want to sent it to a user.
Next time you generate registration keys you should fill the
“Key or KeyID number to start from” with the last
key that you have generated previously. That will ensure that the
generated keys have not been generated before.
Copy a registration code from the file you have just saved.
Now, return to the trial expired page and click
and paste the registration code (if it
wasn't already detected in the clipboard) into the text area from the
Registration page.
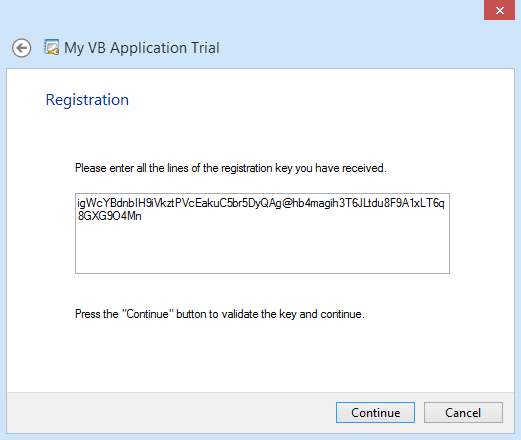
Click to validate the registration key.

Click to exit the Registration Wizard and resume the VB application.
The application is now registered and we can see it in the “About” dialog.

This tutorial will guide you in creating a VB application with licensing and trial support using Advanced Installer.
This walkthrough is designed to help you gain a better understanding of how the licensing feature in Advanced Installer works and how you can implement a licensing component into your own project. Throughout this example we'll create a simple Visual Basic project using Visual Studio 2008.
Our sample application itself will have a simple form but you should find sufficient comments in the underlying code to assist you in implementing this feature into any of your own projects. The project will include a very simple 'About' form which will display the licensed state of the application.
Because the nature of this walkthrough is to help you understand the licensing process in its entirety we are going to flip backwards and forwards between Advanced Installer and the application code. It's assumed that you are familiar with the basics of using Visual Studio so these will not be gone into.
So with our Visual Basic project completed as far as we wish to go at present we'll create a quick build in Advanced Installer using the Import Visual Studio Project wizard and then we will set the licensing options.
- 1. Create the Visual Basic project using Visual Studio
- 2. Use the Advanced Installer Import Wizard for Visual Basic application
- 3. Convert your project to Enterprise
- 4. Add Trial and Licensing
- 5. Choose Trial Options
- 6. Integrate the Licensing Library within application
- 7. Done - Build and install
- 8. Register the application
- 9. Uninstall and Clean-up
1. Create the Visual Basic project using Visual Studio
Using Visual Studio 2008 create a Visual Basic project. Add an menu strip and an About form and save the project.
We will later add the code that will verify the trial period and registration key.
2. Use the Advanced Installer Import Wizard for Visual Basic application
Open Advanced Installer and use the “Visual Studio Application” specialized template.
Complete the wizard by selecting the Visual Basic solution created earlier and you will find your application in Files and Folders page.


3. Convert your project to Enterprise
The Visual Studio Import Wizard created an Advanced Installer Professional project. To use the trial and licensing features we must upgrade the project from Professional to Enterprise. Use the "Project" -> "Options" menu item to display the options page. In the "Project Type" page you will be able to update you project to Enterprise.
4. Add Trial and Licensing
Licensing and trial options can be configured from the Licensing page that you will find in the Tools section of the left pane or alternatively you can access it from the "Installation" -> "Tools" -> "Licensing" menu.

The licensing library will be placed into the Application Folder and you can see it in the Files and Folders page. Note that the name of the library file corresponds with the trial configuration name.
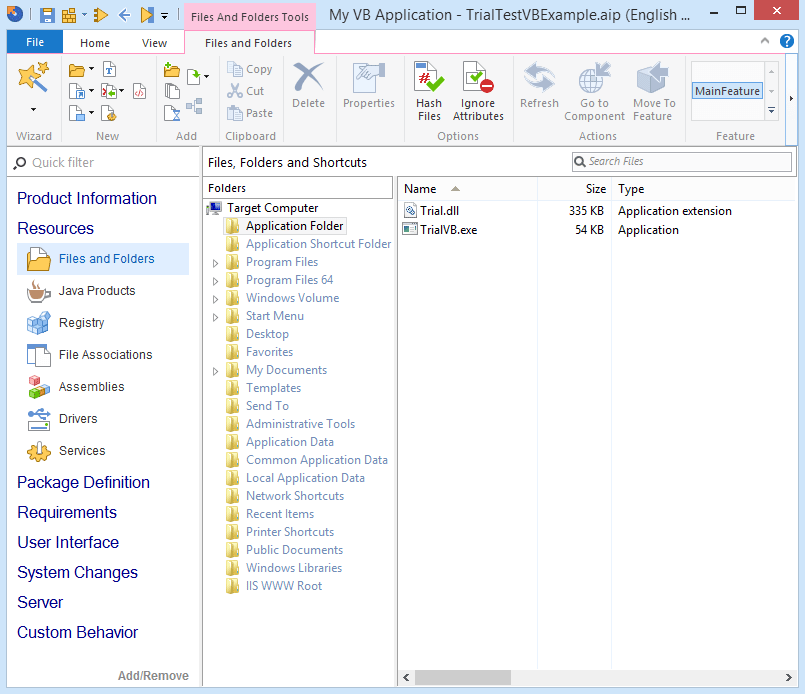

5. Choose Trial Options
The trial options can be found into the Options and Display tab pages of the "Licensing" view. Let's start with the first one.5.1 Trial Options
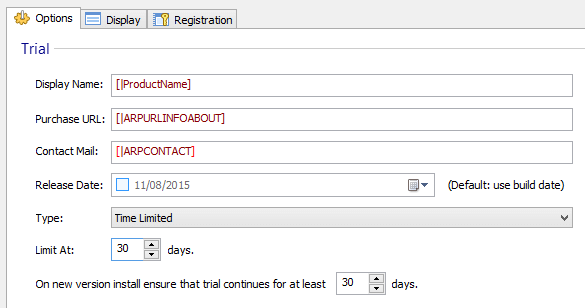
Display Name - the application name which will be used in the Licensing user interface. By default it will be the Product Name you entered during the import wizard or changed in the Product Details page.
Purchase Url - your Web Site page that handles purchases. By default it will be the "Product URL" specified in the Product Details page.
Advanced Installer supports several types of trial periods:
- Time Limited - the trial period will end after a predefined number of days have passed since the installation of the product.
- Uses Limited - the trial period will end after a predefined number of runs of the application.
- Both - the trial period will end when one of the above ends.
Set the trial period quantity into the "Limit At" field to a small value, let's say 10. This way the trial will expire after 10 runs or after 10 days since installation.
Finally in the "On new version install extend to" field we will set the number of days and uses that we are prepared to extend the trial by when a new version of the application is installed. Set this value to 5 because the user already had some time to try the application in the previous version.
Lets not choose to support trial extension options to keep thinks simpler.
Now we should specify which kind of application will use the licensing library.
5.2 Application / Library Type

There are several types of library
- 32-bit ANSI - supports Win9x or higher OS and can't be used in DEP enabled applications
- 32-bit Unicode - supports Win2k or higher OS and can be used in DEP enabled applications
- 64-bit Unicode - supports any 64-bit OS and can be used in DEP enabled applications
5.3 Display Options

Here we can control what our registration wizard will look like and the frequency with which it is displayed during our trial period.

You can change the default “Banner Image” and “Dialog Image” but keep in mind that they will only be used on operating systems earlier than Windows Vista.
5.4 Integration settings summary
There are three settings that we need in order to integrate out VB application with Advanced Installer Licensing:- library name - we will need it to identify which library will be loaded when the application starts
- platform type - we should make sure that our VB application is compatible with the selected platform (32-bit).
- library key - specified in the "Registration" tab page and used when we will call the library functions
6. Integrate the Licensing Library within application
In our Visual Studio project lets set the corresponding platform of the VB application. So into Visual Studio go to "Project" -> "Properties" -> "Compile" -> "Advanced Compile Options" dialog and set the target CPU to x86.Into the Form1.vb source file add the following code snippets:

Imports System Imports System.Runtime.InteropServices ... ' This function does all the workInto the AboutBox1.vb source file add the following code snippets:_ Private Shared Function InitTrial(ByVal aKeyCode As String, ByVal aHWnd As IntPtr) As UInteger End Function ' Use this function to register the application when the application is running _ Private Shared Function DisplayRegistration(ByVal aKeyCode As String, ByVal aHWnd As IntPtr) As UInteger End Function ' The kLibraryKey is meant to prevent unauthorized use of the library. ' Do not share this key. Replace this key with your own from Advanced Installer ' project > Licensing > Registration > Library Key Private Shared kLibraryKey As String = "3F76246B3B0E194506CBC8F512D70B9D0EF8242CEFA92E03237D7152AF70DBD428ED559FBB90" ' The register state of the application which is computed when the form is loaded Private registered As Boolean Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load Try Dim Proc As Process = Process.GetCurrentProcess() ' This call will display the trial dialogs if needed Dim ret = InitTrial(kLibraryKey, Proc.MainWindowHandle) ' 0 return code means that the application is registered using a valid code, otherwise is in trial If ret = 0 Then registered = True End If Catch ex As Exception MessageBox.Show(ex.ToString()) Process.GetCurrentProcess().Kill() End Try End Sub Private Sub DoRegister() Try Dim Proc As Process = Process.GetCurrentProcess() Dim ret = DisplayRegistration(kLibraryKey, Proc.MainWindowHandle) ' 0 return code means that the application was already registered or it ' has just been registered, otherwise is in trial If ret = 0 Then registered = True End If Catch ex As Exception MessageBox.Show(ex.ToString()) Close() End Try End Sub Private Sub AboutToolStripMenuItem_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles AboutToolStripMenuItem.Click Dim dlg As AboutBox1 = New AboutBox1 ' Set the about box member to the registered state computed when the application loaded dlg.registered = Me.registered ' Display the About box dlg.Show() End Sub Private Sub RegisterToolStripMenuItem_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RegisterToolStripMenuItem.Click If registered Then MessageBox.Show("The application is already registered.", "Registered", MessageBoxButtons.OK, MessageBoxIcon.Information) Else DoRegister() End If End Sub
... Public registered As Boolean Private Sub AboutBox1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load ... If Me.registered Then Me.TextBoxDescription.Text = "registered - thank you" Else Me.TextBoxDescription.Text = "trial" End If End SubYou have now successfully integrated the licensing feature into your project.

7. Done - Build and install
Now that we have integrated the licensing functionality lets go back to Advanced Installer and build the installation package.

Congratulations! You have successfully created your licensed application. By default, the application file will be installed under C:\Program Files\Your Company\. Browse to that folder in Windows Explorer and run the application.
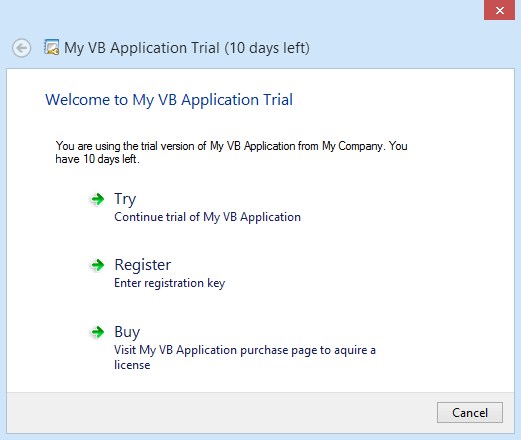

Click the button and the VB form should appear. In the “About” dialog the trial message should be displayed.
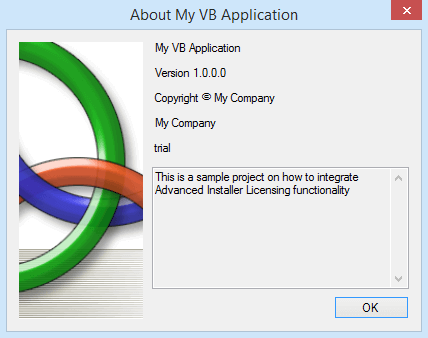
8. Register the application
After 10 tries ( or days - remember the trial type used ) the trial period must expire.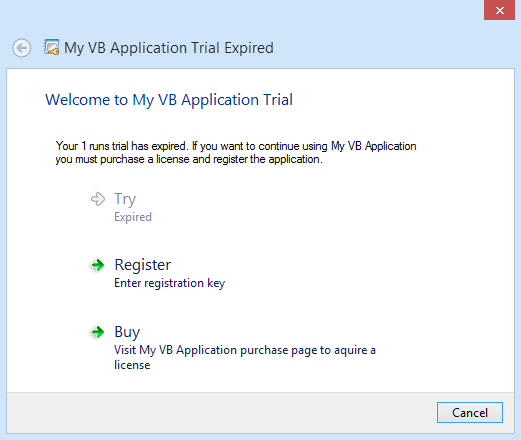
At this point the user needs to register the application since we didn't enable the trial extension option. Registration codes can be generated from the Advanced Installer -> Licensing -> Registration page by using the “Generate Registration Keys” link.
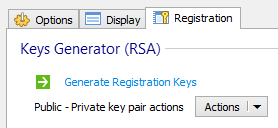
In the “Generate Registry Keys” dialog generate any number of keys you want. The keys will be saved into a file from where you can easily pick one when you want to sent it to a user.

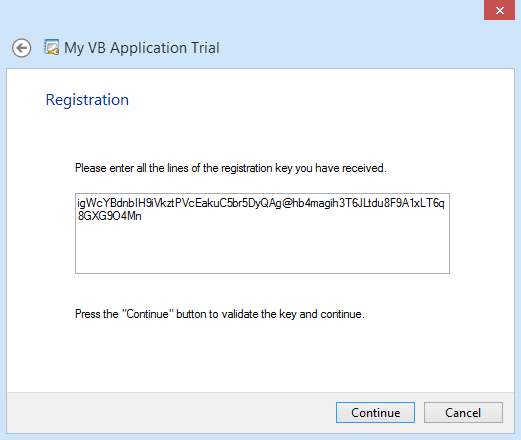
Click to validate the registration key.

Click to exit the Registration Wizard and resume the VB application.
The application is now registered and we can see it in the “About” dialog.

9. Uninstall and Clean-up
During testing or development you may want to remove any trace of the application to reproduce the conditions before the first installation. For that you must:- Uninstall the package - that will delete the installed files.
- Delete the registration code from registry - by default it is saved in Current User\Software\Your Company\Your Product\Registration Key and can be configured from the Advanced Installer -> "Licensing" -> "Registration" page.
- Delete the trial information from the system - in Advanced Installer -> "Licensing" page right-click on the trial configuration and choose "Testing" > "Remove Trial Info" (Advanced Installer must have administrator rights for this operation to succeed).
Comments
Post a Comment